binary_compose<AdaptableBinaryFunction,AdaptableUnaryFunction1,AdaptableUnaryFunction2>
 |
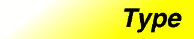 |
 |
|
Categories: functors, adaptors |
Component type: type |
Description
Binary_compose is a function object adaptor.
If f is an Adaptable Binary Function and g1 and g2 are both
Adaptable Unary Functions, and if g1's and g2's return types
are convertible to f's argument types, then binary_compose can be
used to create a function object h such that h(x) is the same as
f(g1(x), g2(x)). [1] [2]
Example
Finds the first element in a list that lies in the range from 1 to 10.
list<int> L;
...
list<int>::iterator in_range =
find_if(L.begin(), L.end(),
compose2(logical_and<bool>(),
bind2nd(greater_equal<int>(), 1),
bind2nd(less_equal<int>(), 10)));
assert(in_range == L.end() || (*in_range >= 1 && *in_range <= 10));
Computes sin(x)/(x + DBL_MIN) for each element of a range.
transform(first, last, first,
compose2(divides<double>(),
ptr_fun(sin),
bind2nd(plus<double>(), DBL_MIN)));
Definition
Defined in the standard header functional, and in the nonstandard
backward-compatibility header function.h. The binary_compose
class is an SGI extension; it is not part of the C++ standard.
Template parameters
Parameter
|
Description
|
Default
|
AdaptableBinaryFunction
|
The type of the "outer" function in the function composition
operation. That is, if the binary_compose is a function object
h such that h(x) = f(g1(x), g2(x)), then AdaptableBinaryFunction
is the type of f.
|
|
AdaptableUnaryFunction1
|
The type of the first "inner" function in the function composition
operation. That is, if the binary_compose is a function object
h such that h(x) = f(g1(x), g2(x)), then AdaptableBinaryFunction
is the type of g1.
|
|
AdaptableUnaryFunction2
|
The type of the second "inner" function in the function composition
operation. That is, if the binary_compose is a function object
h such that h(x) = f(g1(x), g2(x)), then AdaptableBinaryFunction
is the type of g2.
|
|
Model of
Adaptable Unary Function
Type requirements
AdaptableBinaryFunction must be a model of Adaptable Binary Function.
AdaptableUnaryFunction1 and AdaptableUnaryFunction2 must both
be models of Adaptable Unary Function.
The argument types of AdaptableUnaryFunction1 and
AdaptableUnaryFunction2 must be convertible to each other.
The result types of AdaptableUnaryFunction1 and
AdaptableUnaryFunction2 must be convertible, respectively, to
the first and second argument types of AdaptableBinaryFunction.
Public base classes
unary_function<AdaptableUnaryFunction1::argument_type,
AdaptableBinaryFunction::result_type>
Members
Member
|
Where defined
|
Description
|
argument_type
|
Adaptable Unary Function
|
The type of the function object's argument:
AdaptableUnaryFunction::argument_type.
|
result_type
|
Adaptable Unary Function
|
The type of the result: AdaptableBinaryFunction::result_type
|
binary_compose(const AdaptableBinaryFunction& f,
const AdaptableUnaryFunction1& g1,
const AdaptableUnaryFunction1& g2);
|
binary_compose
|
See below.
|
template <class AdaptableBinaryFunction,
class AdaptableUnaryFunction1,
class AdaptableUnaryFunction2>
binary_compose<AdaptableBinaryFunction,
AdaptableUnaryFunction1,
AdaptableUnaryFunction2>
compose2(const AdaptableBinaryFunction&,
const AdaptableUnaryFunction1&,
const AdaptableUnaryFunction2&);
|
binary_compose
|
See below.
|
New members
These members are not defined in the
Adaptable Unary Function
requirements, but are specific to
binary_compose.
Member
|
Description
|
binary_compose(const AdaptableBinaryFunction& f,
const AdaptableUnaryFunction1& g1,
const AdaptableUnaryFunction1& g2);
|
The constructor. Constructs a binary_compose object such that
calling that object with the argument x returns
f(g1(x), g2(x)).
|
template <class AdaptableBinaryFunction,
class AdaptableUnaryFunction1,
class AdaptableUnaryFunction2>
binary_compose<AdaptableBinaryFunction,
AdaptableUnaryFunction1,
AdaptableUnaryFunction2>
compose2(const AdaptableBinaryFunction&,
const AdaptableUnaryFunction1&,
const AdaptableUnaryFunction2&);
|
Creates a binary_compose object. If f, g, and g2 are, respectively,
of classes AdaptableBinaryFunction, AdaptableUnaryFunction1, and
AdaptableUnaryFunction2, then compose2(f, g1, g2) is equivalent to
binary_compose<AdaptableBinaryFunction, AdaptableUnaryFunction1,
AdaptableUnaryFunction2>(f, g1, g2), but is more convenient.
This is a global function, not a member function.
|
Notes
[1]
This is a form of function composition. The unary_compose
adaptor allows composition of Adaptable Unary Functions; note,
however, that once binary functions are introduced, there are several
possible patterns of function composition. The binary_compose
allows you to form a unary function by putting together two unary
functions and a binary function, but you could also, for example,
imagine putting together two unary functions and a binary function to
form a binary function. In that case, f, g1, and g2 would be
combined into a function object h such that h(x,y) = f(g1(x),
g2(y)).
See also
The function object overview, unary_compose,
binder1st, binder2nd.
Copyright ©
1999 Silicon Graphics, Inc. All Rights Reserved.
TrademarkInformation